ReactJS API Integration and Development
In the dynamic landscape of modern web development, ReactJS stands out as a powerful library for building user interfaces. Integrating APIs with ReactJS not only enhances the functionality of web applications but also streamlines data management and user interaction. This article explores the essentials of API integration and development in ReactJS, providing insights and practical tips to optimize your web projects for performance and scalability.
Introduction to ReactJS and API Integration
ReactJS is a popular JavaScript library developed by Facebook for building user interfaces, particularly for single-page applications. It allows developers to create large web applications that can update and render efficiently with changing data. The core concept of ReactJS is the component-based architecture, which enables the reuse of code and simplifies the development process.
- Component-Based Architecture: ReactJS allows developers to build encapsulated components that manage their own state.
- Virtual DOM: ReactJS uses a virtual DOM to improve performance by minimizing direct manipulation of the real DOM.
- Unidirectional Data Flow: ReactJS follows a unidirectional data flow, making it easier to debug and understand.
API integration in ReactJS involves connecting the application to external data sources or services. This is crucial for building dynamic applications that rely on real-time data. By using APIs, developers can fetch, update, and manipulate data from external sources seamlessly. ReactJS provides various methods like Fetch API and Axios for making HTTP requests to integrate APIs efficiently. Understanding how to integrate APIs with ReactJS is essential for creating robust and scalable applications.
Setting up Your Development Environment
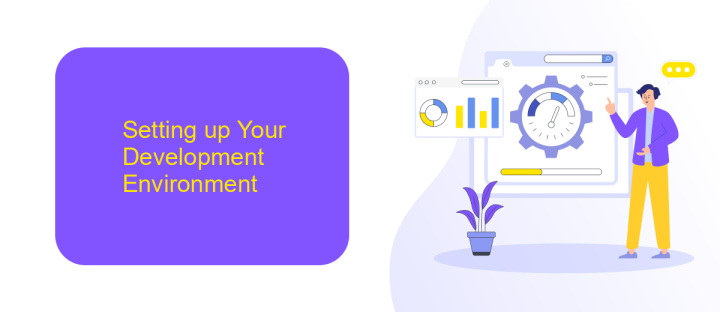
To begin developing with ReactJS, you'll need to set up a robust development environment. Start by installing Node.js and npm, which are essential for managing packages and running React applications. Once installed, use the command line to create a new React project with Create React App, a tool that simplifies the setup process. This will generate a project structure with all the necessary files and dependencies, allowing you to focus on development rather than configuration.
For seamless API integration, consider using services like ApiX-Drive, which can automate data transfer between your React application and various APIs. This tool simplifies the process of connecting different services, saving you time and effort in manual coding. Additionally, ensure you have a code editor like Visual Studio Code, which offers extensions and features tailored for JavaScript and React development. With these tools in place, you'll be well-equipped to start building dynamic and responsive applications with ReactJS, leveraging powerful APIs to enhance functionality.
Making API Requests with Fetch API or Axios
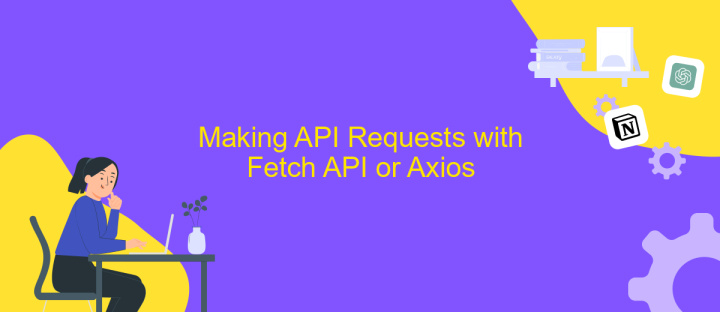
When integrating APIs in a ReactJS application, making HTTP requests is a fundamental task. Two popular methods for handling these requests are using the Fetch API and Axios. Both tools allow developers to communicate with external services and retrieve data, but they have distinct features and benefits.
- Fetch API is a built-in JavaScript function that provides a simple interface for fetching resources asynchronously. It returns a promise, making it easy to handle asynchronous operations with then() and catch() methods.
- Axios is a third-party library that simplifies HTTP requests. It supports older browsers and provides features like request cancellation, automatic JSON data transformation, and interceptors for handling requests and responses.
Choosing between Fetch API and Axios depends on your project requirements. Fetch is great for basic requests and has no dependencies, while Axios offers more advanced features and is easier to use for complex requests. Ultimately, both tools are effective for making API requests in ReactJS, allowing developers to build dynamic and responsive applications.
Handling API Responses and Data Management
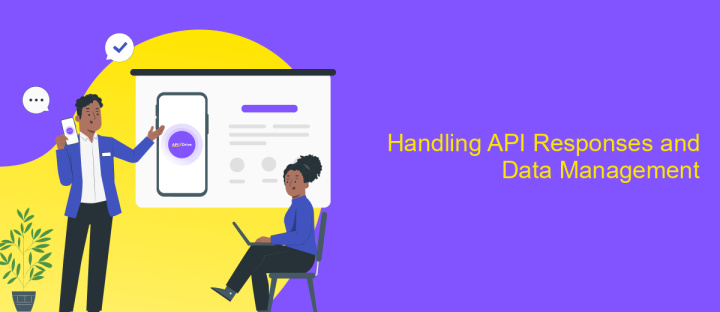
Handling API responses effectively is crucial in ReactJS applications to ensure seamless user experiences. When an API request is made, the response can vary in structure and content, necessitating robust handling mechanisms. The first step in managing these responses is to parse the data correctly, often using JSON parsing methods, to convert the raw response into a usable format.
Once the data is parsed, developers must implement error handling to manage potential issues such as network failures or unexpected response formats. This can be achieved through try-catch blocks or by using promises with .catch() methods. Proper error handling ensures that the application remains stable and provides meaningful feedback to users.
- Parse JSON responses to extract relevant data.
- Implement error handling to manage unsuccessful requests.
- Utilize state management tools like Redux or Context API for data storage.
- Ensure data consistency by normalizing API responses.
Efficient data management in ReactJS involves utilizing state management libraries such as Redux or Context API. These tools help maintain a consistent state across the application, allowing components to access and update data as needed. By normalizing API responses, developers can ensure that data structures remain consistent, reducing redundancy and improving application performance.

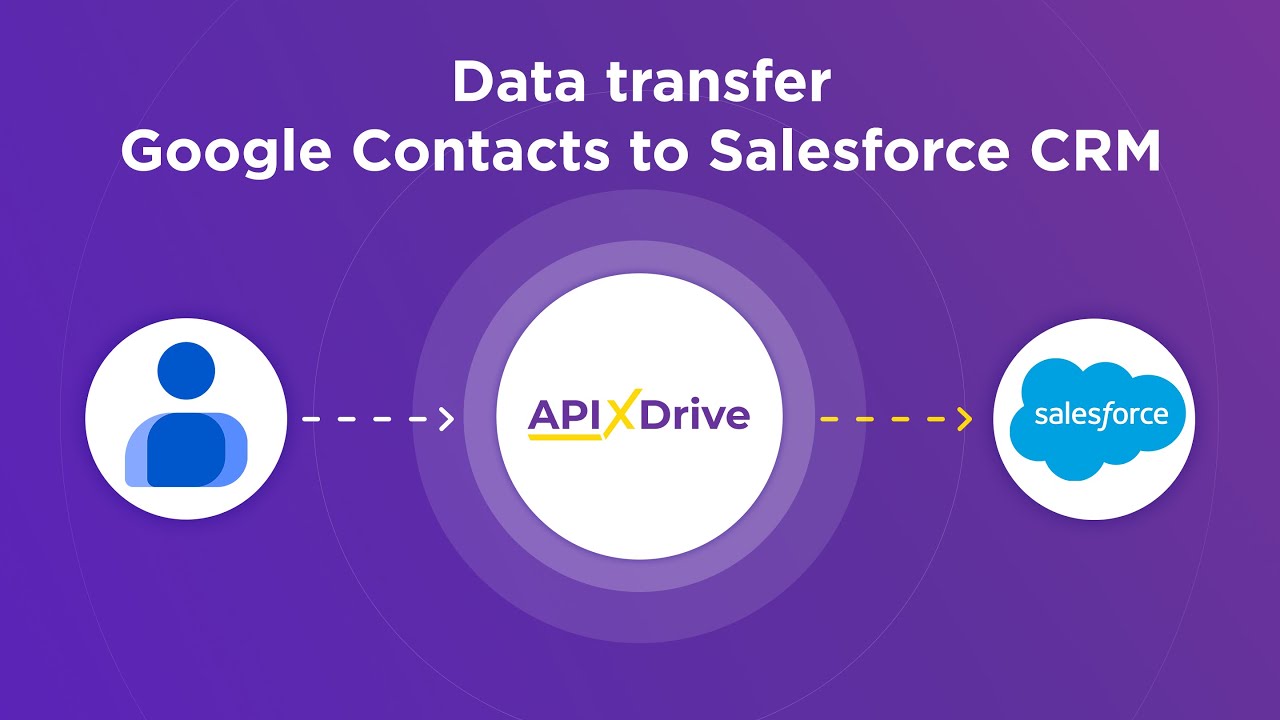
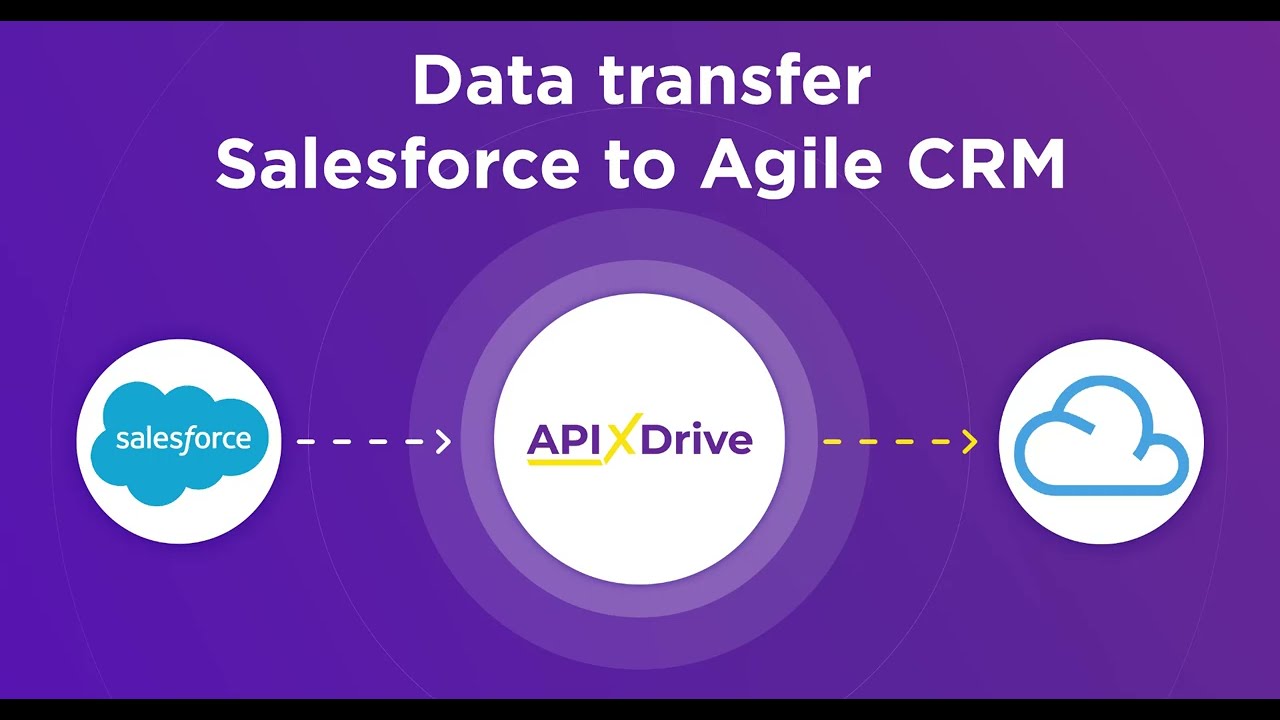
Best Practices and Advanced Concepts
When integrating APIs with ReactJS, it's crucial to adhere to best practices to ensure efficient and maintainable code. Begin by organizing your codebase with a clear separation of concerns. Use custom hooks to manage API requests, which can help in reusing logic across components. Implement error handling and loading states to enhance user experience. Utilizing environment variables for storing API keys and endpoints is a good security practice. Furthermore, consider using libraries like Axios for more streamlined HTTP requests and better error management.
Diving into advanced concepts, explore the use of React Query or SWR for efficient data fetching and caching. These libraries can significantly enhance performance by reducing redundant requests and keeping data fresh. For more complex integrations, services like ApiX-Drive can simplify the process by automating data exchange between different platforms. This is particularly useful for scaling applications with multiple API integrations. Lastly, always keep an eye on API rate limits and optimize your requests accordingly to prevent throttling and ensure smooth application performance.
FAQ
What is ReactJS, and why is it popular for API integration and development?
How can I fetch data from an API in a ReactJS application?
What are some best practices for integrating APIs with ReactJS?
How can I automate and streamline API integrations in my ReactJS projects?
How can I ensure my ReactJS application is secure when integrating with APIs?
Time is the most valuable resource for business today. Almost half of it is wasted on routine tasks. Your employees are constantly forced to perform monotonous tasks that are difficult to classify as important and specialized. You can leave everything as it is by hiring additional employees, or you can automate most of the business processes using the ApiX-Drive online connector to get rid of unnecessary time and money expenses once and for all. The choice is yours!