MySQL C++ Connector API
The MySQL C++ Connector API serves as a robust interface for developers seeking to integrate MySQL databases with C++ applications. This powerful API facilitates seamless database connectivity, enabling efficient data manipulation and retrieval. By leveraging this connector, developers can harness the full potential of MySQL's capabilities within their C++ projects, ensuring high performance, reliability, and scalability. Explore its features to enhance your application's database interactions and streamline development processes.
Introduction
The MySQL C++ Connector API is an essential tool for developers looking to integrate MySQL databases with C++ applications. It offers a comprehensive set of functionalities that allow seamless interaction with MySQL databases, enabling developers to execute queries, retrieve data, and manage database connections efficiently. This API is designed to enhance productivity by providing a robust and reliable interface that simplifies database operations within C++ environments.
- Easy integration with C++ applications
- Support for both synchronous and asynchronous operations
- Comprehensive documentation and community support
- Compatibility with various MySQL versions
- Secure and efficient database connection management
Utilizing the MySQL C++ Connector API can significantly reduce development time and effort, as it abstracts the complexities involved in database interactions. By leveraging its features, developers can focus on building the core functionalities of their applications, ensuring that database operations are handled smoothly and securely. Whether you are developing a small-scale application or a large enterprise system, the MySQL C++ Connector API offers the flexibility and performance needed to meet your database connectivity requirements.
Prerequisites
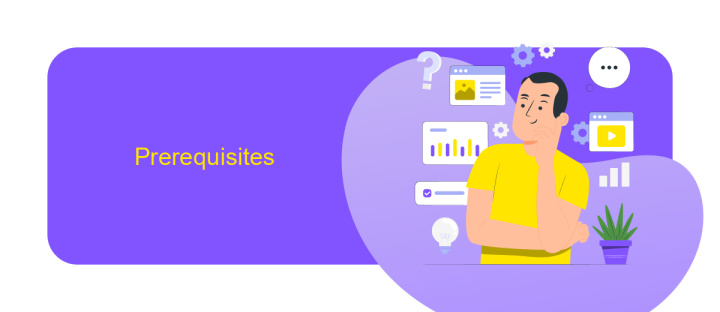
Before diving into using the MySQL C++ Connector API, ensure that you have a fundamental understanding of C++ programming and basic SQL queries. Familiarity with database concepts such as tables, indexes, and relationships will be beneficial. Additionally, having a MySQL server installed and running on your local machine or accessible remotely is crucial. This setup will allow you to test and execute your database operations seamlessly.
It is also important to have a development environment configured for C++ development. Tools like Visual Studio or Code::Blocks can be useful for writing and compiling your code. Furthermore, make sure you have the MySQL C++ Connector installed, which can be downloaded from the official MySQL website. For those looking to integrate MySQL databases with other applications, consider using services like ApiX-Drive. This platform can simplify the integration process by automating data transfers and synchronizations, making it easier to manage your database interactions efficiently.
API Overview
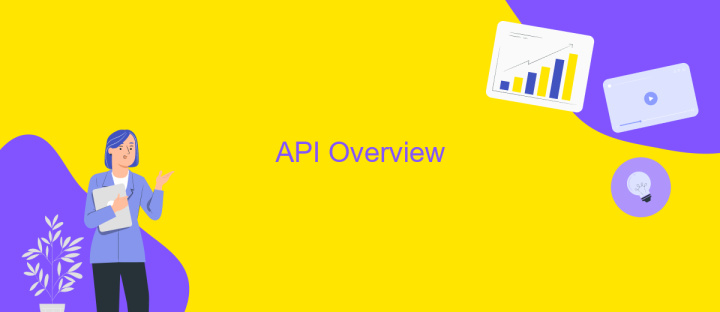
The MySQL C++ Connector API provides developers with a robust and efficient way to interact with MySQL databases using C++. It offers a set of classes and functions that simplify the process of connecting to a database, executing queries, and retrieving results. The API is designed to be intuitive and easy to use, making it an ideal choice for both novice and experienced developers who need to integrate MySQL database functionality into their C++ applications.
- Connection Management: Establish and manage connections to MySQL databases with ease.
- Query Execution: Execute SQL queries and statements efficiently.
- Result Handling: Retrieve and process results from database queries seamlessly.
- Error Handling: Robust error detection and handling mechanisms.
- Prepared Statements: Support for executing parameterized queries to enhance security and performance.
By leveraging the MySQL C++ Connector API, developers can ensure that their applications are both powerful and scalable. The API's comprehensive features allow for seamless integration with MySQL databases, enabling the development of high-performance applications. Whether you're building a small project or a large-scale enterprise application, the MySQL C++ Connector API offers the tools and functionality necessary to meet your database interaction needs effectively.
Connecting to a Database
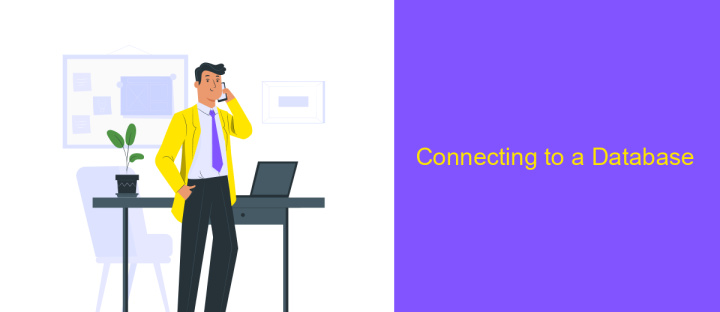
To establish a connection to a MySQL database using the C++ Connector API, you first need to ensure that the necessary libraries are correctly installed and configured. This involves setting up the development environment with the MySQL Connector/C++ and ensuring that your compiler can locate the required header files and libraries. Once the setup is complete, you can proceed to establish a connection to the database.
Begin by including the appropriate headers in your C++ program. You'll need to create a `sql::Driver` instance to interact with the MySQL server. Subsequently, utilize the `sql::Connection` object to initiate the actual connection process. This connection requires specifying the database server's address, the username, and the password.
- Include necessary headers: `mysql_driver.h` and `mysql_connection.h`.
- Create a `sql::Driver` instance using `get_driver_instance()`.
- Establish a connection using the `sql::Connection` object.
- Specify the server address, username, and password.
Once connected, you can execute SQL queries and retrieve results. Ensure to handle exceptions that may arise during the connection process, such as incorrect credentials or network issues. Proper error handling will help maintain a robust application and provide informative feedback to the user.

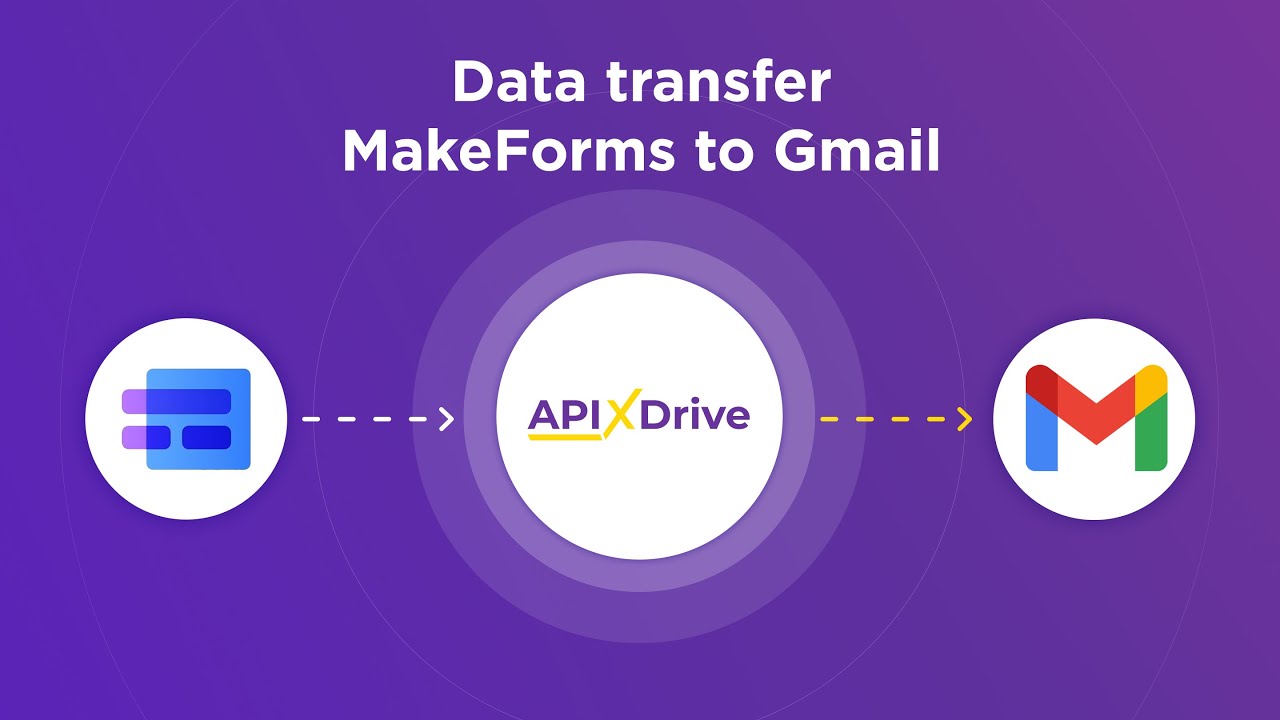
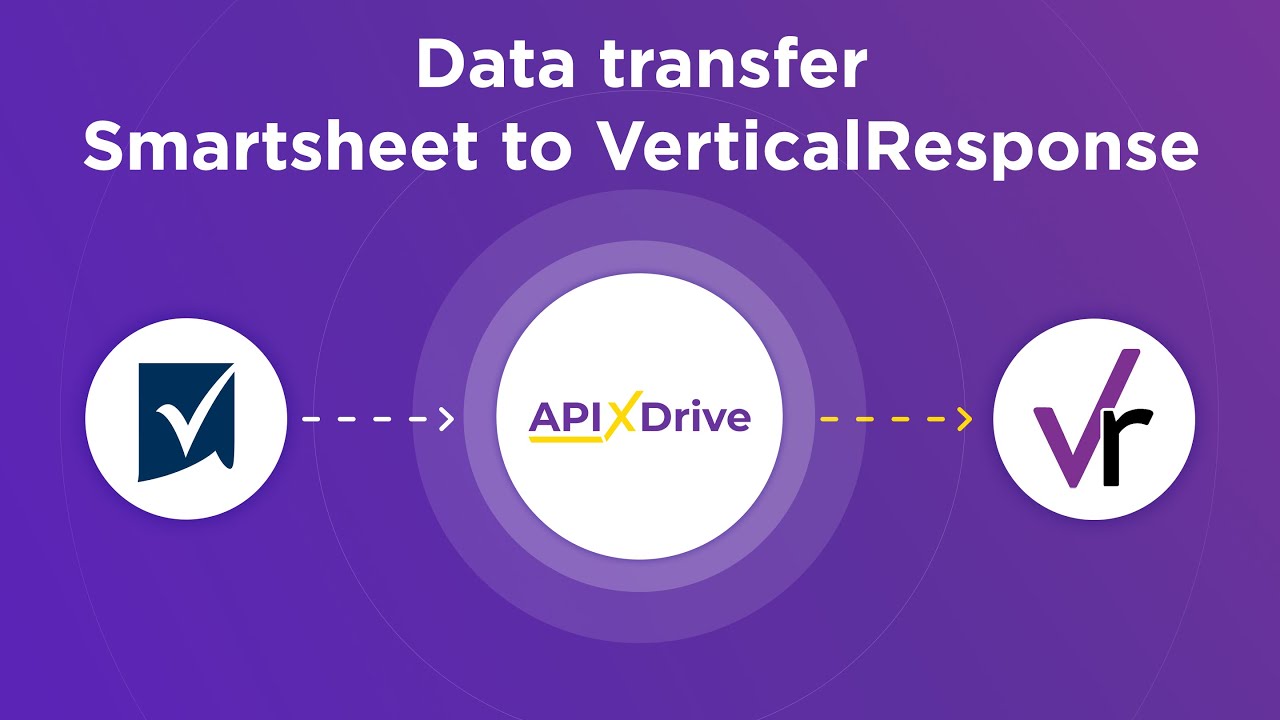
Executing Queries and Retrieving Results
Executing queries in MySQL using the C++ Connector API involves creating a connection to the database and preparing SQL statements. Once connected, you can use the `executeQuery` method to run SQL commands. This method returns a `ResultSet` object that contains the data retrieved from the database. To handle this data, you can iterate over the `ResultSet` using a loop, accessing each row and column through methods like `getString`, `getInt`, and others, depending on the data type.
Retrieving results efficiently requires understanding of how to manage the `ResultSet` lifecycle. It's important to close the `ResultSet` and any `Statement` objects after use to free resources. For those integrating MySQL with other applications, services like ApiX-Drive can streamline the process by automating data transfer between systems. This can be particularly useful when dealing with large volumes of data or when real-time updates are necessary. By leveraging such tools, developers can enhance the efficiency and reliability of their database interactions.
FAQ
What is the MySQL C++ Connector API used for?
How do I install the MySQL C++ Connector?
Can I use MySQL C++ Connector with any version of MySQL?
How can I handle database connection errors in MySQL C++ Connector?
How can I automate integration of MySQL C++ Connector with other services?
Apix-Drive is a simple and efficient system connector that will help you automate routine tasks and optimize business processes. You can save time and money, direct these resources to more important purposes. Test ApiX-Drive and make sure that this tool will relieve your employees and after 5 minutes of settings your business will start working faster.